The Oder of Method Execution in Java
Understanding the flow of program execution when dealing with method in Java
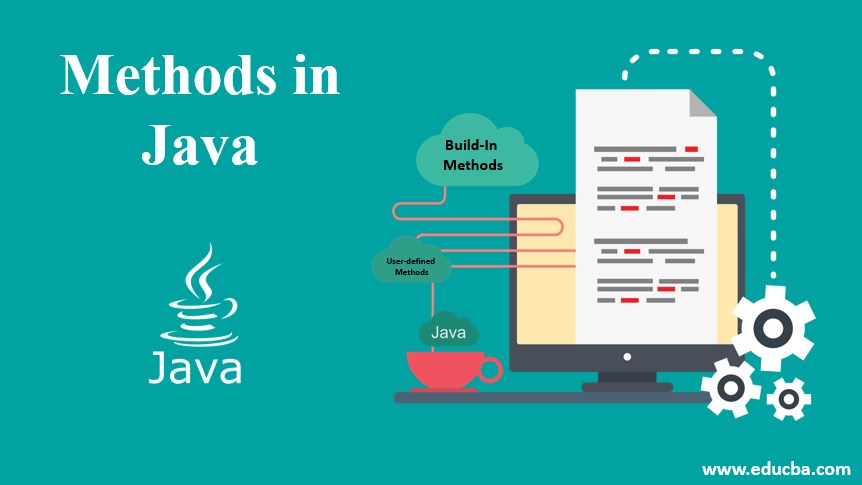
The Order of Execution in Java
The order of execution in Java is linear, it start from top to bottom. But, with functional approach we can change this order. Functional programming is a programming paradigm which allow programmers to create software into reusable modules called methods or functions.
Method in Java is a block codes that execute instructions written within the body of the method when invoke or call, a method can return a value or might not return any value. A method can take input aka argument, but it most be define parameter in its parameter-list when creating it. More about methods in Java.
Here I will show how to create a static methods in Java, and how to nested methods within method. I will also show you how we can change the order of execution using methods in Java.
Let’s first create a normal Java Application with just our normal main
method:
public class Main {
public static void main(String[] args) {
String myName = "Alieu Saidy";
System.out.println("Hello, my name is " +myName);
int age = 12;
System.out.println("I am " +age+ " old");
String designation = "Student";
System.out.println("I am a " +designation+ " at Hands on Academy");
}
}
Output:
Hello, my name is Alieu Saidy
I am 12 old
I am a Student at Hands on Academy
In the above example the execute starting from top to bottom. The first print statement execute first and moves all the way to the bottom. This is the normal flow execution in programming, in this all the logics are stack.
Changing the Order of Execution
Let’s see how we change that. Well, it is pretty simple all we need to do to create static method(s) and invoke them in a way I want. What are you saying?
public class Main {
public static void main(String[] args) {
// invoke myName method
myName();
}
// method that will display name
static void myName(){
String myName = "Alieu Saidy";
System.out.println("Hello, my name is " +myName);
}
// method that will display age
static void myAge(){
int age = 12;
System.out.println("I am " +age+ " old");
}
// method that will display designation
static void myDesignation(){
String designation = "Student";
System.out.println("I am a " +designation+ " at Hands on Academy");
}
}
Output:
Hello, my name is Alieu Saidy
In the above example I have re-factored the code by creating methods and write the related business logic in their respective methods, then I invoked only one method which is myName()
method to show how power functional programming can be.
Now let’s begin invoking all the methods we defined in a sequence we want:
- First, want to print the designation of the person to the console so I invoke the method that defines that
- Second, I want to print the name of the person to the console so I invoke the method that defines the name.
- Finally, I invoke the method that defines age.
Let’s refactor our code once more:
public class Main {
public static void main(String[] args) {
// invoke myDesignation
myDesignation();
// invoke myName
myName();
// invoke myAge
myAge();
}
// method that will display name
static void myName(){
String myName = "Alieu Saidy";
System.out.println("My name is " +myName);
}
// method that will display age
static void myAge(){
int age = 12;
System.out.println("I am " +age+ " old");
}
// method that will display designation
static void myDesignation(){
String designation = "Student";
System.out.println("I am a " +designation+ " at Hands on Academy");
}
}
Output:
I am a Student at Hands on Academy
My name is Alieu Saidy
I am 12 old
Well, I hope your question is answered now. I invoked the method in the specific order I want.
Let’s discuss more the order of execution in these regards because the last block of code happens to be executed first. How did that happen?
As we know the main method is the entry point of any Java Application. When I run/execute my program the compiler will focus its attention on the main method, it will execute the first statement it found in our case which is myDesignation()method. When a method is invoked or called the reference is passed to the compiler, the compiler will pause the execution on that particular line then go wherever that method is defined within your program and execute whatever is defined within its body then proceed with the normal flow(which linear execution line after line) which is the next preceding line. The execution of a method doesn’t depends on where they are created in most case, but rather where they are called or invoked.
With our above example, the myDesignation()
is the last method we defined but happens to be the first to be executed.
A Method Calls Other Methods
In programming is possible for a method to call other methods or even pass other methods as an argument if they return a value. Let’s create another method below the Mydesignation()
method call myInfo
package order_execution;
public class Main {
public static void main(String[] args) {
// invoke myInfo
myInfo();
}
// method that will display name
static void myName(){
String myName = "Alieu Saidy";
System.out.println("My name is " +myName);
}
// method that will display age
static void myAge(){
int age = 12;
System.out.println("I am " +age+ " old");
}
// method that will display designation
static void myDesignation(){
String designation = "Student";
System.out.println("I am a " +designation+ " at Hands on Academy");
}
// this method will invoke all the above methods
static void myInfo(){
myName(); // call myName method
myAge(); // call myAge method
myDesignation(); // call myDesignation method
}
}
Output:
My name is Alieu Saidy
I am 12 old
I am a Student at Hands on Academy
Wow myInfo() method has called other methods and what is defined in them got executed upon calling myInfo() method. You have seen how power is this programming approach could be. With a functional approach we can decide which code to execute before the other.
Conclusion
A method is a spice of code which execute a specific instruction when call or invoke, a method returns a value or may not return any value to the caller, and a method can take an optional input called an argument when a parameter is defined. Functional programming is a programming approach which focuses on separating business logic into reusable modules called methods. Methods can change the order of execution in a program, the method can also call or methods, and they will be executed along.